Filter results
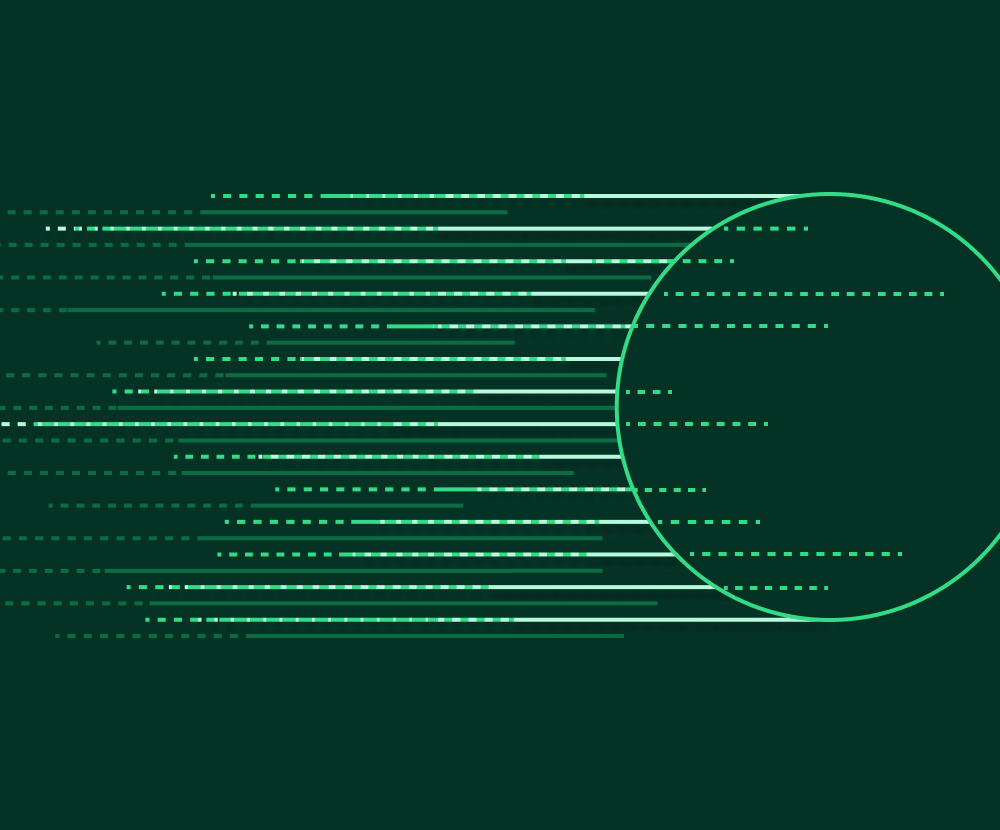
May 29, 2024
6 min read
Making Terabytes of Data Queryable Within Seconds to Drive Fast Decision-Making
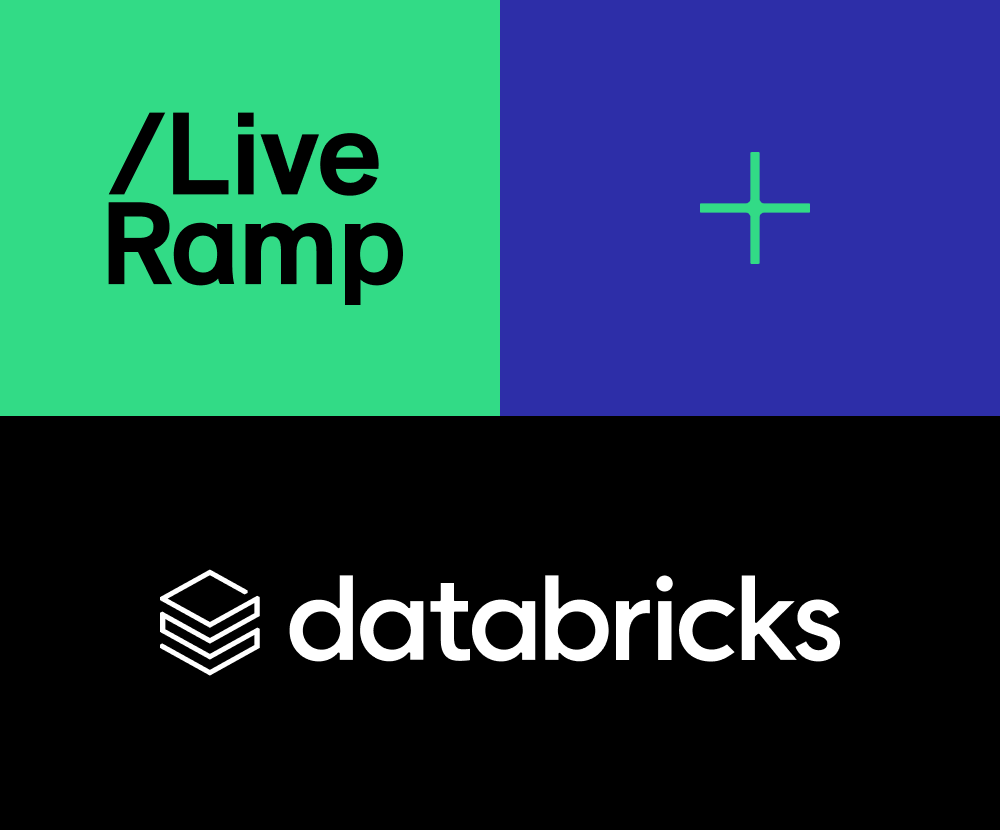
Feb 26, 2024
5 min read
Deliver Next-Generation Customer Experiences with LiveRamp Identity in Databricks Marketplace
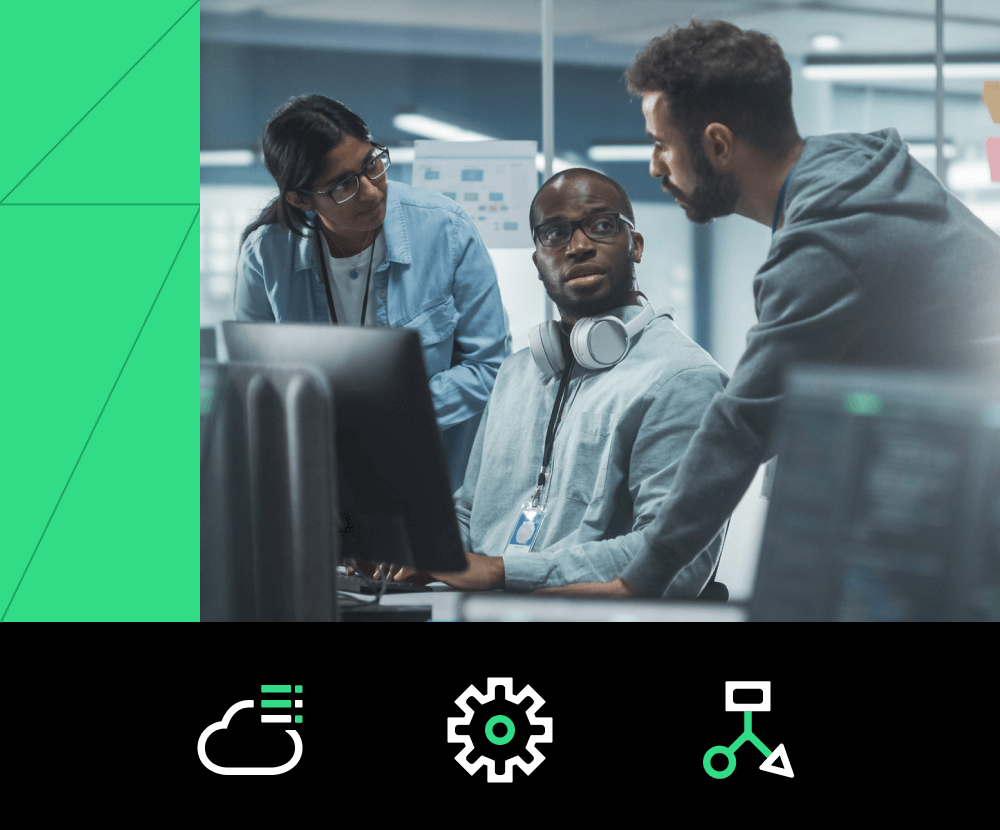
Jan 26, 2024
5 min read
Deploying Opentelemetry (OTEL) Agent to Your GCE Instances
Subscribe for Updates
Stay up to date with the latest from LiveRamp.